Components
Textfield
Used to enter spesific text input form
Component Status Details
Status component contains a list of checks and completeness that has been tested and owned by each component
Textfields allow user input. The border should light up simply and clearly indicating which field the user is currently editing.
Legion has 5 basic types of textfield:
- Basic Text Field
- Text Field with Label
- Text Field with Helper
- Required Text Field
- Optional Text Field
Legion has 5 states of textfield:
- State Normal
- Error State
- Success State
- Disable State
- Locked State
You can also implement prefix and suffix for your project.
For textfield customization, Legion provides 6 types of icon for the textfield:
- Left Icon
- Right Icon
- Prefix with Left Icon
- Prefix with Right Icon
- Suffix with Left Icon
- Suffix with Right Icon
And also Legion has 5 variants :
- Password Field
- Date Field
- Time Field
- Dropdown Field
- Unit Field
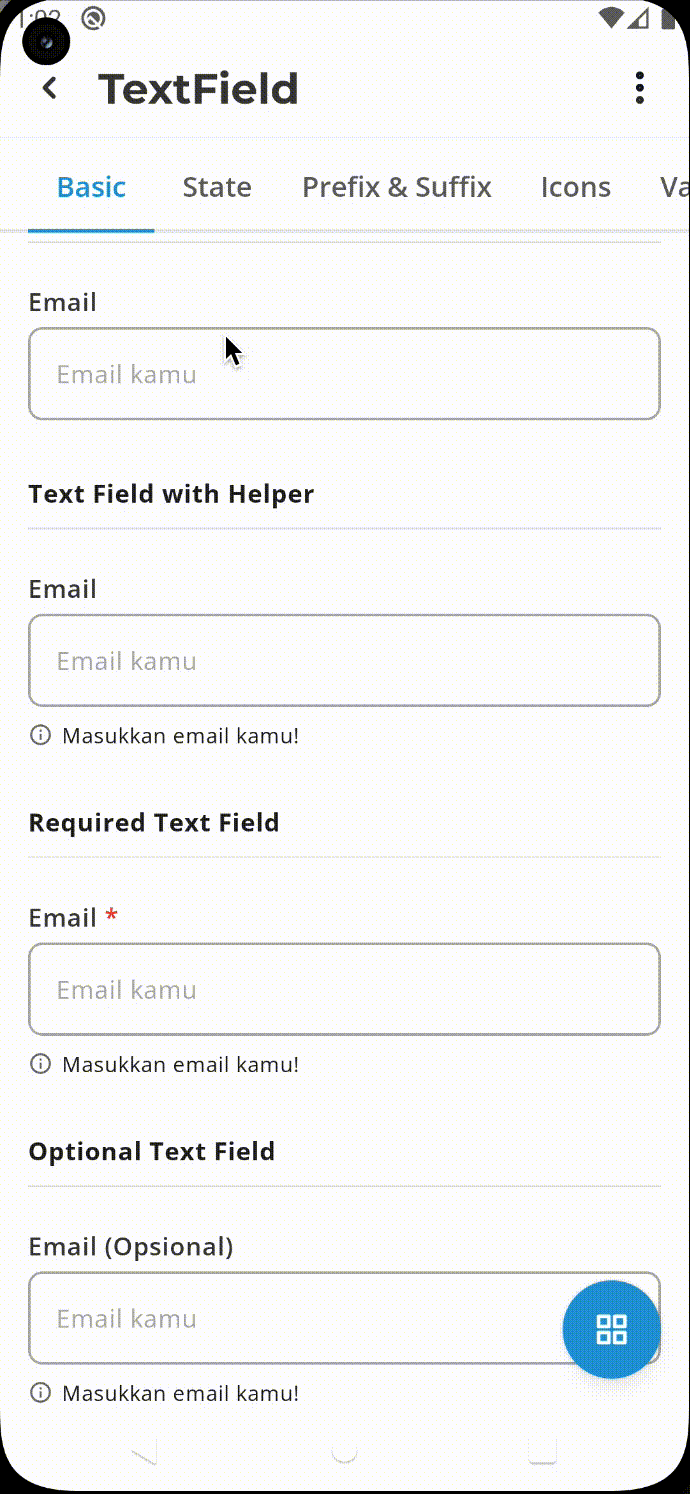
Usage
Basic text field
Static in Xml
<com.telkom.legion.component.edittext.LgnEditText// define your attribute's here... />
Programatically
...LgnEditText(requireContext()).apply {// Your View's customization here}...
Text Field with Label
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...android:hint="Email".../>
Programatically
...LgnSingleField(requireContext()).apply {hint = "Email"}...
Text Field with Helper
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:helperText="Masukkan email kamu!".../>
Programatically
...LgnSingleField(requireContext()).apply {helper = "Masukkan email kamu"}...
Required Text Field
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:isRequired="true".../>
Programatically
...LgnSingleField(requireContext()).apply {isRequired = true}...
Optional Text Field
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:isOptional="true".../>
Programatically
...LgnSingleField(requireContext()).apply {isOptional = true}...
State Normal
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField// define your attribute's here... />
Programatically
...LgnSingleField(requireContext()).apply {//Your View's customization here}...
Error State
Programatically
...LgnSingleField(requireContext()).apply {error = "Email sudah digunakan!"}...
Success State
Programatically
...LgnSingleField(requireContext()).apply {succcess = "Email dapat digunakan!"}...
Disable State
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...android:enabled="false".../>
Programatically
...LgnSingleField(requireContext()).apply {isEnabled = true}...
Locked State
Programatically
...LgnSingleField(requireContext()).apply {isLocked = true}...
Prefix Text
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:prefixText="Email".../>
Programatically
...LgnSingleField(requireContext()).apply {prefixText = "email"}...
Suffix Text
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:suffixText="\@telkom.co.id".../>
Programatically
...LgnSingleField(requireContext()).apply {suffixText = "email"}...
Left Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:startIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {startIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Right Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:endIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {endIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Prefix with Left Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:prefixStartIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {prefixStartIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Prefix with Right Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:prefixEndIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {prefixEndIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Suffix with Left Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:suffixStartIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {suffixStartIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Suffix with Right Icon
Static in Xml
<com.telkom.legion.component.textfield.LgnSingleField...app:suffixEndIconDrawable="@drawable/ic_calendar".../>
Programatically
...LgnSingleField(requireContext()).apply {suffixEndIconDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.ic_calendar)}...
Password Field
Static in Xml
<com.telkom.legion.component.textfield.LgnPasswordField// define your attribute's here... />
Programatically
...LgnPasswordField(requireContext()).apply {// Your View's customization here}...
Date Field
Static in Xml
<com.telkom.legion.component.textfield.LgnCalendarField...app:dateFormat="FULL_DATE".../>
Programatically
...LgnCalendarField(requireContext()).apply {dateFormat = DateFormat.DEFAULT_DATE}...
Time Field
Static in Xml
<com.telkom.legion.component.textfield.LgnTimeField// define your attribute's here... />
Programatically
...LgnTimeField(requireContext()).apply {// Your View's customization here}...
Dropdown Field
To use dropdown, first define widget or component in your xml.
<com.telkom.legion.component.textfield.LgnDropdownField// define your attribute's here... />
And then put dropdown items in your code.
...etDropDown.addAll(items)...
Unit Field
To use Unit Field, first define widget or component in your xml.
<com.telkom.legion.component.textfield.LgnSingleUnitField// define your attribute's here... />
And then put dropdown items in your code.
...etUnit.addAll(items)...
Attributes
Attribute Name | Xml Attrs | Related method(s) | Description |
---|---|---|---|
Hint | android:hint | text | To show hint on textfield |
Placeholder text | app:placeholderText | placeHolder | To show placeholder on textfield |
Helper text | app:helperText | helper | To show helper text directly via xml |
Digits | app:digits | N/A | To show digit number on textfield |
Input Type ¹ | android:inputType | inputType | To set input type on text field directly via xml |
Enable Status | android:enabled | isEnable | To set enable or disable text area directly via xml |
Required Status | app:isRequired | isRequired | To set required status on text area directly via xml |
Optional Status | app:isOptional | isOptional | To set optional status on text area directly via xml |
Suffix Text ¹ | app:suffixText | suffixText | To set suffix text on text field |
Prefix Text ¹ | app:prefixText | prefixText | To set prefix text on text field |
Override Dropdown Behaviour ⁴ ⁵ | app:overrideDropdown | overrideDropdown | To override default behaviour of dropdown |
Prefix End Icon Drawable ¹ | app:prefixEndIconDrawable | prefixEndIconDrawable | To set icon on behind text prefix on text field directly via xml |
Prefix Start Icon Drawable ¹ | app:prefixStartIconDrawable | prefixStartIconDrawable | To set icon on front text prefix on text field directly via xml |
Suffix End Icon Drawable ¹ | app:suffixEndIconDrawable | suffixEndIconDrawable | To set icon on behind text suffix on text field directly via xml |
Suffix Start Icon Drawable ¹ | app:suffixStartIconDrawable | suffixStartIconDrawable | To set icon on front text suffix on text field directly via xml |
Ellipsize Placement ¹ | android:ellipsize | ellipsize | To set where Ellipsize on text field directly via xml |
Max Lines ¹ | android:maxLines | maxLines | To set maximum lines on text field directly via xml |
Max Length ¹ | android:maxLength | maxLength | To set max length on text field directly via xml |
Locked Field ¹ | N/A | isLocked | To set locked field on LgnSingleField |
Get Edit Text Instance | N/A | editText | To get edit text instance from component |
Add Values ⁴ ⁵ | N/A | addAll(List<String>) | To set list of string to dropdown on component |
Add Click Listener ⁴ ⁵ | N/A | setOnClickListener | To set click listener on dropdown if you want override default behaviour |
Add Dropdown Listener ⁴ | N/A | setListener | To set listener on dropdown when value changed |
Add Unit Listener ⁵ | N/A | setOnUnitListener | To set listener on dropdown on unit field when value changed |
Get Selected Unit Value ⁵ | N/A | unit | To get selected unit on unit field |
Get Edit Text on Dropdown Field Instance ⁵ | N/A | dropDown | To get edittext on dropdown field instance on unit field |
Set Error State | N/A | isError | To set text field to error state |
Set Error Message | N/A | error | To set message on error state on text field, if you set error message, text field will automaticly configure to error state |